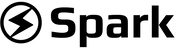
Beta
Statically-typed interpreted language
Table of contents
Prerequisites
Download
Changelog
Beta 0.1.1 (2022-08-09)
-
Fixed the addShape and deleteShape functions of the ppt library.
Implementation documentation
Table of contents
Implementation guide
This guide will help you implement the Spark Compiler in your projects (systems, tools, games, etc.).
​
Before we start, make sure you downloaded and imported all dependencies from the prerequisites section.
​
-
Download the Spark Compiler ZIP file from the download section.
-
Import the files inside the ZIP file to your VBA project. These are the class files Spark.cls, SparkCore.cls, SparkLexer.cls, SparkParser.cls, SparkInterpreter.cls, and SparkNativeLibs.cls. The first one is the main and only one you'll be using directly.
-
In order to compile and run a Spark file we use the CompileFile and Run functions, respectively. Note that you are required to compile a file before you can run it. See the example below on how to compile and run a Spark file. By default the Run function will call the main function in the code and you can change it to some other function. Spark code files use the .spk extension.
1
2
3
Dim Spark As New Spark
Spark.CompileFile "C:\Spark projects\hello_world.spk" ' Compile the file's code
Spark.Run ' Run the code (executes the "main" function)
Logging
Output is sent to the OnLog event. For example the print function from the io library sends output.
1
2
3
4
5
6
7
8
9
10
11
Dim WithEvents Spark As Spark
​
Sub Run()
Set Spark = New Spark
Spark.CompileFile "C:\Spark projects\hello_world.spk" ' Compile the file's code
Spark.Run ' Run the code (executes the "main" function)
End Sub
​
Sub Spark_OnLog(Msg As String)
Debug.Print Msg ' Print the log to the immediate window
End Sub
Error handling
Errors are handled through the OnError event and the error message is given by the ErrMsg parameter. After the event is fired, all code execution is stopped, because an End command is issued.
1
2
3
4
5
6
7
8
9
10
11
Dim WithEvents Spark As Spark
​
Sub Run()
Set Spark = New Spark
Spark.CompileFile "C:\Spark projects\hello_world.spk" ' Compile the file's code
Spark.Run ' Run the code (executes the "main" function)
End Sub
​
Sub Spark_OnError(ErrMsg As String)
Debug.Print ErrMsg ' Handle the error by printing it to the immediate window
End Sub
Native Libraries
Spark comes with 5 libraries called Native Libraries which include basic functionality from math to string manipulation to controling PowerPoint. However, sometimes you may not want users to have full control and disable some libraries. That's why there's a SetNativeLibrary function which allows you to enable or disable any native library.
1
2
3
4
Dim Spark As New Spark
Spark.SetNativeLibrary "ppt", False ' Disables the "ppt" native library
Spark.CompileFile "C:\Spark projects\hello_world.spk" ' Compile the file's code
Spark.Run ' Run the code (executes the "main" function)
Custom libraries
You can import other Spark files, but sometimes you wan to add new functionality or have more control using the VBA programming language. Therefore you can make and then load your own Spark libraries, just like the native libraries. Follow the steps below to start making your very own library.
​
-
Create a class which will hold all your library information and functions.
-
Add a function called GetDefinitions that returns a string. This function should return all definitions (written in Spark syntax) of each constant and function that your library exports. Each definition should be separated by a semicolon.
-
Add your library's functions and add the prefix sparklib_ to their name.
​
In the end your class should look something like the example below.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
Public Function GetDefinitions() As String
GetDefinitions = _
"const int NEWLINE = 13;" & _
"void alert(string message);" & _
"float getSlideWidth();" & _
"float getSlideHeight();"
End Function
​
Public Sub sparklib_alert(Message As String)
MsgBox Message, vbExclamation, "Alert"
End Sub
​
Public Function sparklib_getSlideWidth() As Single
sparklib_getSlideWidth = ActivePresentation.SlideShowWindow.Width
End Function
​
Public Function sparklib_getSlideHeight() As Single
sparklib_getSlideHeight = ActivePresentation.SlideShowWindow.Height
End Function
Now in order to distribute your library you have to export the class as a file. To use the library you simple load it using the LoadLibrary function.
1
2
3
4
Dim Spark As New Spark
Spark.LoadLibrary "test", New TestLib ' loads the library using the library class
Spark.CompileFile "C:\Spark projects\custom_lib_test.spk" ' Compile the file's code
Spark.Run ' Run the code (executes the "main" function)
Finally to use the library, you simply import it by its ID.
1
2
3
4
5
import "test";
​
void main() {
alert("hi");
}
Language reference documentation
Table of contents
"Hello" example
1
2
3
4
5
6
7
// Spark example code
​
import "io";
​
void main() {
print("Hello Spark!\n");
}
Syntaxes
If statement
if (condition)
consequent
[else
alternate]
While loop
while (condition) {
statements
}
Do while loop
do {
statements
} while (condition);
For loop
for (statement; expression; statement) {
statements
}
For-each loop
for (statement : expression) {
statements
}
Switch statement
switch (discriminant) {
[case test:
consequent
[...]]
[default:
consequent]
}
Single-line comment
// comment
Multi-line comment
/* comment */
Function definition
type name([type name[, type name ...]]) {
statements
}
Function call
function([argument[, argument ...]]);
Variable declaration
[private|public] [const] type name[[][[] ...]][ = init][, name[[][[] ...]][ = init] ...];
Variable assignment
variable[[index][[index] ...]] = value;
Throw error
throw errorMessage;
Array length
len(array)
Copy array items
copy(array)
Type casting
(type)value
Import native library
import library;
Import Spark file
import file;
Binary operation
left binaryOperator right
Unary operation
unaryOperator value
Array/String access
array|string[index][[index] ...]
Conditional ternary operator
condition ? expressionIfTrue : expressionIfFalse
Array builder
type[size][[size] ...]
Number type suffix
number<l|L|f|F|d|D>
​
l/L - long
f/F - float
d|D - double
Default number type: Integer
Binary operators
-
Addition (+)
-
Subtraction (-)
-
Multiplication (*)
-
Division (/)
-
Remainder (%)
-
Bitwise AND (&)
-
Bitwise OR (|)
-
Bitwise XOR (^)
-
Logical AND (&&)
-
Logical OR (||)
-
Left shift (<<)
-
Right shift (>>)
-
Unsigned right shift (>>>)
-
Exponentiation (**)
-
Equality (==)
-
Inequality (!=)
-
Less than (<)
-
Less than or equal (<=)
-
Greater than (>)
-
Greater than or equal (>=)
-
Addition assignment (+=)
-
Subtraction assignment (-=)
-
Multiplication assignment (*=)
-
Division assignment (/=)
-
Multiplication assignment (*=)
-
Remainder assignment (%=)
-
Bitwise AND assignment (&=)
-
Bitwise OR assignment (|=)
-
Bitwise XOR assignment (^=)
-
Logical AND assignment (&&=)
-
Logical OR assignment (||=)
-
Left shift assignment (<<=)
-
Right shift assignment (>>=)
-
Unsigned right shift assignment (>>>=)
-
Exponentiation assignment (**=)
Unary operators
-
Plus (+)
-
Minus (-)
-
Logical NOT (!)
-
Increment (++)
-
Decrement (--)
-
Type of (typeof)
Data types
Name
Keyword
Size
Range
Integers
Byte
Short
Integer
Long
byte
short
int
long
1 bytes
2 bytes
4 bytes
8 bytes
0 – 255
-32,768 – 32,767
-2,147,483,648 – 2,147,483,647
​-9,223,372,036,854,775,808 – 9,223,372,036,854,775,807
Floats
Float
Double
float
double
4 bytes
8 bytes
-3.402823E38 – -1.401298E-45; 1.401298E-45 – 3.402823E38
-1.79769313486231E308 – -4.94065645841247E-324; 4.94065645841247E-324 – 1.79769313486232E308
Other
Boolean
String
Any
bool
string
any
2 bytes
–
–
false or true
–
–
Native libraries
-
Input/output (io)
-
const ​short FATTR_NORMAL = 0
-
const ​short FATTR_READ_ONLY = 1
-
const ​short FATTR_HIDDEN = 2
-
const ​short FATTR_SYSTEM = 4
-
const ​short FATTR_VOLUME = 8
-
const ​short FATTR_DIRECTORY = 16
-
const ​short FATTR_ALIAS = 64
-
void print(any... text)​
-
void println(any... text)​
-
string readTextFile(string path, string charset = "utf-8")
-
void writeTextFile(string path, string content, string charset = "utf-8")
-
void deleteFile(string path)
-
void renameFile(string path, string newPath)
-
bool fileExists(string path)
-
string dir(string pathName = "", short attributes = 0)
-
-
System (sys)
-
int msgbox(string prompt, int buttons, string title)
-
float timer()
-
int doEvents()
-
bool isKeyPressed(int keyCode)
-
-
Math (math)
-
double sin(double value)
-
double cos(double value)
-
double tan(double value)
-
double atn(double value)
-
double abs(double value)
-
int floor(double value)
-
int ceil(double value)
-
int round(double value)
-
int sign(double value)
-
double sqrt(double value)
-
int parseInt(string value)
-
double parseFloat(string value)
-
double min(double a, double b)
-
double max(double a, double b)
-
double random()
-
-
String (string)
-
​string toString(any value)​​
-
int strLen(string s)
-
string[] split(string s, string delimiter)
-
string join(string[] arr, string delimiter = "")
-
short getCharCode(string char)
-
string getCharFromCode(short charCode)
-
string substr(string s, int start, int length = -1)
-
string substring(string s, int start, int end = -1)
-
bool startsWith(string s, string searchString, int pos = 0)
-
bool endsWith(string s, string searchString, int endPos = -1)
-
-
PowerPoint (ppt)
-
const ​short SHAPE_RECTANGLE = 1
-
const ​short SHAPE_ISOSCELES_TRIANGLE = 7
-
const ​short SHAPE_OVAL = 9
-
void addShape(int slideIndex, short shapeType, string shapeName, float left, float top, float width, float height)
-
void setShapeLeft(int slideIndex, string shapeName, float left)
-
void setShapeTop(int slideIndex, string shapeName, float top)
-
void setShapeWidth(int slideIndex, string shapeName, float width)
-
void setShapeHeight(int slideIndex, string shapeName, float height)
-
void setShapeRotation(int slideIndex, string shapeName, float rotation)
-
void setShapeText(int slideIndex, string shapeName, string text)
-
void setShapeFillColor(int slideIndex, string shapeName, int color)
-
int getShapeFillColor(int slideIndex, string shapeName)
-
void setShapeFillTransparency(int slideIndex, string shapeName, float transparency)
-
float getShapeFillTransparency(int slideIndex, string shapeName)
-
void setShapeFillPicture(int slideIndex, string shapeName, string pictureFile)
-
void setShapeLineColor(int slideIndex, string shapeName, int color)
-
int getShapeLineColor(int slideIndex, string shapeName)
-
void setShapeLineTransparency(int slideIndex, string shapeName, float transparency)
-
float getShapeLineTransparency(int slideIndex, string shapeName)
-
float getShapeLeft(int slideIndex, string shapeName)
-
float getShapeTop(int slideIndex, string shapeName)
-
float getShapeWidth(int slideIndex, string shapeName)
-
float getShapeHeight(int slideIndex, string shapeName)
-
float getShapeRotation(int slideIndex, string shapeName)
-
void setShapeAction(int slideIndex, string shapeName, short mouseActivation, short action)
-
void setShapeActionHyperlinkAddress(int slideIndex, string shapeName, short mouseActivation, string hyperlinkAddress)
-
void setShapeActionRunMacro(int slideIndex, string shapeName, short mouseActivation, string macroName)
-
string getShapeText(int slideIndex, string shapeName)
-
string getShapeName(int slideIndex, int shapeIndex)
-
void deleteShape(int slideIndex, string shapeName)
-
void duplicateShape(int slideIndex, string shapeName)
-
int getShapeCount(int slideIndex)
-
int getSlideCount()
-
int getCurrentSlide()
-
string[] getShapes(int slideIndex)
-
void gotoSlide(int slideIndex)
-
If statement
If condition evaluates to true then consequent code is executed, if evalauted to false then alternate code is executed.
if (condition)
consequent
else
alternate
Examples
int x = 0;
if (x == 0)
println("It's zero");
else
println("It's not zero");
int x = 0;
bool isZero;
if (x == 0) {
println("It's zero");
isZero = true;
} else {
println("It's not zero");
isZero = false;
}
While loop
While the condition evaluates to true then consequent code keeps being executed in a loop.
while (condition) {
consequent
}
Examples
int i = 0;
while (i < 4) {
print(i++);
}
​
// Output: 0123
int i = 4;
do {
print(i++);
} while (i < 4);
​
// Output: 4